Python Tuple Modify Element
It is not possible to modify or update an element of a tuple since tuples are immutable. If we want to modify the element of a tuple, we have to create a new tuple with a new value in the position of the modified element. Let's take 'x' is the existing tuple and 'y' is the new tuple. First of all, copy the elements of 'x' from 0th position to pos-2 position into 'y' as: y = x[0:pos-1] Concatenate the new element to the new tuple 'y'. Thus the new element is stored in the position of the element being modified. y = y+new Now concatenate the remaining elements from 'x' by eliminating the element which is at 'pos-1'. It means we should concatenate elements from 'pos' till the end. The total tuple can be assigned again to the old name 'x'. x = y+x[pos:]
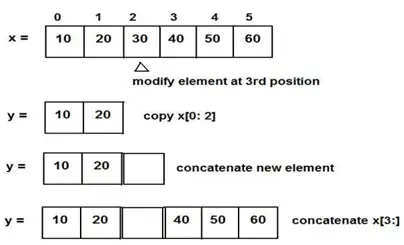
Tuple Modify element Example:
A Python program to modify or replace an existing element of a tuple with a new element.
#modifying an existing element of a tuple
num = (10, 20, 30, 40, 50)
print(num) #accept new element and position number
lst= [int(input('Enter a new element:'))]
new = tuple(lst)
pos = int(input('Enter position no:'))
num1 = num[0:pos-1]
num1 = num1+new
#concatenate the remaining elements of num from pos till end
num = num1+num[pos:]
print(num)