Python Object reference
In the languages like C and Java, when we pass values to a function, we think about two ways:
- Pass by value or call by value
- Pass by reference or call by reference
Pass by value represents that a copy of the variable value is passed to the function and any modifications to that value will not reflect outside the function.Pass by reference represents sending the reference or memory address of the variable to the function. The variable value is modified by the function through memory address and hence the modified value will reflect outside the function also.
Neither of these two concepts is applicable in Python . In Python, the values are sent to functions by means of object references.We know everything is considered as an object in Python. All numbers are objects, strings are objects, and the datatypes like tuples, lists, and dictionaries are also objects.
pass by object value
A Python program to pass an integer to a function and modify it.
def modify(x):
x=15
print(x, id(x))
x= 10
modify(x)
print(x, id(x))
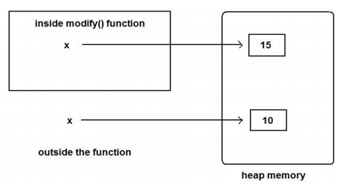
pass by object reference
In Python integers, floats, strings and tuples are immutable . That means their data cannot be modified . When we try to change their value, a new object is created with the modified value. On the other hand, lists and dictionaries are mutable . That means, when we change their data, the same object gets modified and new object is not created.
A Python program to pass a list to a function and modify it.
def modify(lst):
lst.append(9)
print(lst, id(lst))
lst = [1,2,3,4]
modify(lst)
print(lst, id(lst))
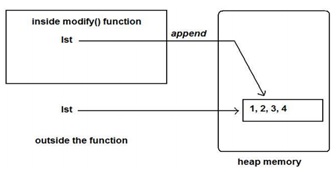
A caution is needed here. If we create altogether a new object inside the function, then it will not be available outside the function. To understand this, we will rewrite the Program
A Python program to create a new object inside the function does not modify outside object.
def modify(lst):
lst = [10, 11, 12] #to create a new list
print(lst, id(lst))
lst = [1,2,3,4]
modify(lst)
print(lst, id(lst))
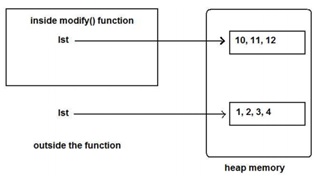