Python Anonymous(Lambda) Functions
A function without a name is called 'anonymous function' . So far, the functions we wrote were defined using the keyword 'def'.But anonymous functions are not defined using 'def'. They are defined using the keyword lambda and hence they are also called 'Lambda functions' . Let's take a normal function that returns square of a given value.
def square(x):
return x*x
The same function can be written as anonymous function as:
lambda x: x*x
The format of lambda functions is:
lambda argument_list: expression
Normally, if a function returns some value, we assign that value to a variable as:
y = square(5)
But, lambda functions return a function and hence they should be assigned to a function as:
f = lambda x: x*x
Here, 'f' is the function name to which the lambda expression is assigned. Now, if we call the function f() as:
value = f(5)
Now, 'value' contains the square value of 5, i.e. 25
A Python program to create a lambda function that returns a square value of a given number.
f = lambda x: x*x
value = f(5)
print('Square of 5 =', value)
Lambda functions contain only one expression and they return the result implicitly. Hence we should not write any 'return' statement in the lambda functions.
Add two numbers using Lambda.
f = lambda x, y: x+y
result = f(1.55, 10)
print('Sum =', result)
A lambda function to find the bigger number in two given numbers.
max = lambda x, y: x if x>y else y
a, b = [int(n) for n in input("Enter two numbers: ").split(',')]
print('Bigger number =', max(a,b))
Because a lambda functions is always represented by a function, we can pass a lambda function to another function. It means we are passing a function (i.e. lambda) to another function. This makes processing the data very easy. For example, lambda functions are generally used with functions like filter(), map() or reduce().
Lambda with filter() Function
The filter() function is useful to filter out the elements of a sequence depending on the result of a function. We should supply a function and a sequence to the filter() function as:
filter(function, sequence)
Here, the 'function' represents a function name that may return either True or False; and 'sequence' represents a list, string or tuple. The 'function' is applied to every element of the 'sequence' and when the function returns True, the element is extracted otherwise it is ignored. Before using the filter() function, let's first write a function that tests whether a given number is even or odd.
def is_even(x):
if x%2==0:
return True
else:
return False
Now, we can use this function inside filter() to test the elements of a list 'lst' as: filter(is_even, lst)
A Python program using filter() to filter out even numbers from a list.
def is_even(x):
if x%2==0:
return True
else:
return False
lst = [10, 23, 45, 46, 70, 99]
lst1 = list(filter(is_even, lst))
print(lst1)
Passing lambda function to filter() function is more elegant.
A lambda that returns even numbers from a list.
lst = [10, 23, 45, 46, 70, 99]
lst1 = list(filter(lambda x: (x%2 == 0), lst))
print(lst1)
Lambda with map()
Function The map() function is similar to filter() function but it acts on each element of the sequence and perhaps changes the elements. The format of map() function is:
map(function, sequence)
The 'function' performs a specified operation on all the elements of the sequence and the modified elements are returned which can be stored in another sequence.
A Python program to find squares of elements in a list.
def squares(x):
return x*x
lst = [1, 2, 3, 4, 5]
lst1 = list(map(squares, lst))
print(lst1)
A lambda function that returns squares of elements in a list.
lst = [1, 2, 3, 4, 5]
lst1 = list(map(lambda x: x*x, lst))
print(lst1)
It is possible to use map() function on more than one list if the lists are of same length. In this case, map() function takes the lists as arguments of the lambda function and does the operation. For example,
map(lambda x, y: x*y, lst1, lst2)
Here, lambda function has two arguments 'x' and 'y'. Hence, 'x' represents 'lst1' and 'y' represents 'lst2'. Since lambda is showing x*y, the respective elements from lst1 and lst2 are multiplied and the product is returned.
A Python program to find the products of elements of two different lists using lambda function.
lst1 = [1, 2, 3, 4, 5]
lst2 = [10, 20, 30, 40, 50]
lst3 = list(map(lambda x, y: x*y, lst1, lst2))
print(lst3)
Lambda with reduce() function
The reduce() function reduces a sequence of elements to a single value by processing the elements according to a function supplied. The reduce() function is used in the format:
reduce(function, sequence)
For example, we write the reduce() function with a lambda expression, as:lst = [1, 2, 3, 4, 5]
reduce(lambda x, y: x*y, lst)
The lambda function is taking two arguments and returning their product. Hence, starting from the 0th element of the list 'lst', the first two elements are multiplied and the product is obtained. Then this product is multiplied with the third element and the product is obtained. Again this product is multiplied with the fourth element and so on. The final product value is returned, as shown in Figure
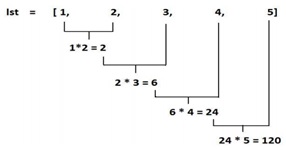
from functools import *
lst = [1, 2, 3, 4, 5]
result = reduce(lambda x, y: x*y, lst)
print(result)