Python count number of words
We have len() function that returns the number of char acters in a string. Suppose we want to find the length of a string without using len() function, we can use a for loop as:
i=0 for s in str: i+=1
This for loop repeats once for each character of the string 'str'. So, if the string has 10 characters, the for loop repeats for 10 times. Hence, by counting the number of repetitions, we can find the number of characters. This is done by simply incrementing a counting variable 'i' inside the loop as shown in the preceding code.
A Python program to find the length of a string without using len() function.
s = input('Enter a string:')
c=0
for i in s:
c+=1
print('\nNo. of characters:', c)
A Python program to find number of words in a given string:
s= "Digi Brains Academy is a Software Training Institute"
print ("The original string is : " + s)
res = len(s.split())
print ("The number of words in string are : ",res)
s= "Digi Brains Academy is a Software Training Institute"
c=0
for i in s:
if i==' ':
c=c+1
print('No of Words: ',c+1)
Inserting Sub String into a String
Let's take a string with some characters. In the middle of the string, we want to insert a sub string. This is not possible because of immutable nature of strings. But, we can find a way for this problem.
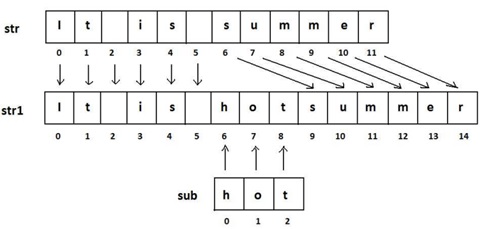
Count words in string example
A Python program to insert a sub string in a string in a particular position.
str = input('Enter a string:')
sub = input('Enter a sub string:')
n = int(input('Enter position no:'))
n-=1
l1 = len(str)
l2 = len(sub)
str1 = []
for i in range(n):
str1.append(str[i])
for i in range(l2):
str1.append(sub[i])
for i in range(n, l1):
str1.append(str[i])
str1 = ''.join(str1)
print(str1)