Python Aliasing and Cloning Lists
List aliasing
Giving a new name to an existing list is called 'aliasing'. The new name is called 'alias name'. For example, take a list 'x' with 5 elements as
x = [10, 20, 30, 40, 50]
To provide a new name to this list, we can simply use assignment operator as:
y = x
In this case, we are having only one list of elements but with two different names 'x' and 'y'. Here, 'x' is the original name and 'y' is the alias name for the same list. Hence, any modifications done to 'x' will also modify 'y' and vice versa. Observe the following statements where an element x[1] is modified with a new value.
x = [10,20,30,40,50]
y = x #x is aliased as y
print(x) will display [10,20,30,40,50]
print(y) will display [10,20,30,40,50]
x[1] = 99 #modify 1st element in x
print(x) will display [10,99,30,40,50]
print(y) will display [10,99,30,40,50]
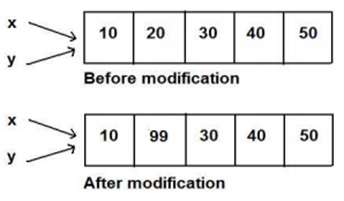
List cloning
If the programmer wants two independent lists, he should not go for aliasing. On the other hand, he should use cloning or copying.
Obtaining exact copy of an existing object (or list) is called 'cloning'. To clone a list, we can take help of the slicing operation as:
y = x[:] #x is cloned as y
When we clone a list like this, a separate copy of all the elements is stored into 'y'. The lists 'x' and 'y' are independent lists. Hence, any modifications to 'x' will not affect 'y' and vice versa. Consider the following statements:
x = [10,20,30,40,50] y = x[:] #x is cloned as y print(x) will display [10,20,30,40,50] print(y) will display [10,20,30,40,50] x[1] = 99 #modify 1st element in x print(x) will display [10,99,30,40,50] print(y) will display [10,20,30,40,50]
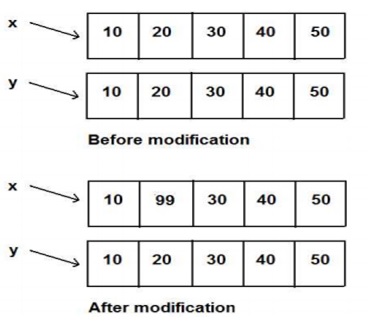
We can observe that in cloning, modifications to a list are confined only to that list. The same can be achieved by copying the elements of one list to another using copy() method. For example, consider the following statement:
y = x.copy() #x is copied as y
When we copy a list like this, a separate copy of all the elements is stored into 'y'. The lists 'x' and 'y' are independent. Hence, any modifications to 'x' will not affect 'y' and vice versa.