Python Immutable strings
An immutable object is an object whose content cannot be changed. On the other hand, a mutable object is an object whose content can be changed as and when required. In Python, numbers, strings and tuples are immutable. Lists, sets, dictionaries are mutable objects.
s1='one'
s2='two'
print(id(s1))
print(id(s2))
s2 = s1
print(id(s1) )
print(id(s2))
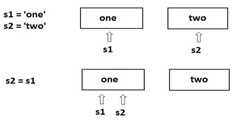
Identity number of an object internally refers to the memory address of the object and is given by id() function. If we display identity numbers using id() function, we can find that the identity number of 's2' and 's1' are same since they refer to one and the same object.
Replacing a String with another String
The replace() method is useful to replace a sub string in a string with another sub string. The format of using this method is:
stringname.replace(old, new)
This will replace all the occurrences of 'old' sub string with 'new' sub string in the main string.
str = 'That is a beautiful bird'
s1 = 'bird'
s2 = 'flower'
str1 = str.replace(s1, s2)
print(str1)
Splitting and Joining Strings
The split() method is used to brake a string into pieces. These pieces are returned as a list. For example, to brake the string 'str' where a comma (,) is found, we can write:
str.split(',')
Observe the comma inside the parentheses. It is called separator that represents where to separate or cut the string. Similarly, the separator will be a space if we want to cut the string at spaces. In the following example, we are cutting the string 'str' wherever a comma is found. The resultant string is stored in 'str1' which is a list.
str = 'one,two,three,four'
str1 = str.split(',')
print(str1)
A Python program to accept and display a group of numbers.
str = input('Enter numbers separated by space:')
lst = str.split('')
for i in lst:
print(i)
When a group of strings are given, it is possible to join them all and make a single string. For this purpose, we can use join() method as: separator.join(str)
where, the 'separator' represents the character to be used between the strings in the output. 'str' represents a tuple or list of strings. In the following example, we are taking a tuple 'str' that contains 3 strings as:
str = ('one', 'two', 'three')
We want to join the three strings and form a single string. Also, we want to use hyphen (-) between the three strings in the output. The join() method can be written as:
str = ('one', 'two', 'three')
str1 = "-".join(str)
print(str1)
str = ['apple', 'guava', 'grapes', 'mango']
sep =':'
str1 = sep.join(str)
print(str1)