Python numpy arrays
numpy is a package that contains several classes, functions, variables etc. to deal with scientific calculations in Python. numpy is useful to create and also process single and multi-dimensional arrays. In addition, numpy contains a large library of mathematical functions like linear algebra functions and Fourier transforms.
Install numpy arrays
To work with numpy, we should first import numpy module into our Python programs as:
import numpywe can use any of the objects from that package. But, to refer to an object we should use the format:numpy.object .
Installing numpypip install numpy
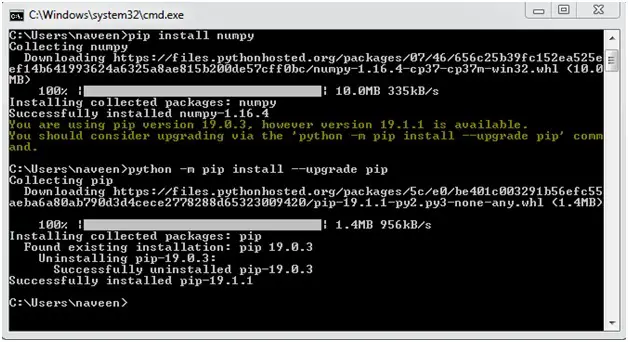
creation of numpy array examples:
Python program to create a simple array using numpy.
Copied
import numpy
arr = numpy.array([10, 20, 30, 40, 50])
print(arr)
Second version of Program to create an array.
Copied
import numpy as np
arr = np.array([10, 20, 30, 40, 50])
print(arr)
Third version of Program to create an array.
Copied
from numpy import *
arr = array([10, 20, 30, 40, 50])
print(arr)
Creating arrays in numpy can be done in several ways. Some of the important ways are:
- Using array() function
- Using linspace() function
- Using logspace() function
- Using arange() function
- Using zeros() and ones() functions