Python Tuple Insert Element
Since tuples are immutable, we cannot modify the elements of the tuple once it is created. Now, let's see how to insert a new element into an existing tuple. Let's take 'x' as an existing tuple. Since 'x' cannot be modified, we have to create a new tuple 'y' with the newly inserted element. First of all, copy the elements of 'x' from 0th position to pos-2 position into 'y' as:
y = x[0:pos-1]
Concatenate the new element to the new tuple 'y'.
y = y+new
Concatenate the remaining elements (from pos-1 till end) of x to the new tuple 'y'. The total tuple can be stored again with the old name 'x'.
x = y+x[pos-1:]
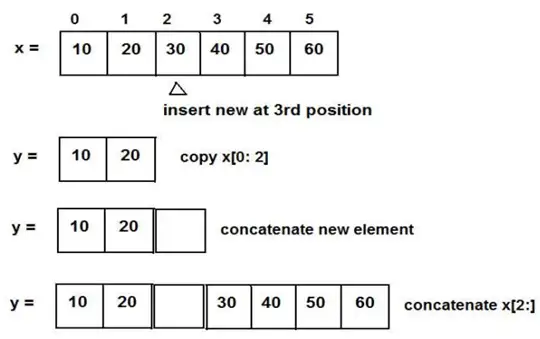
Tuple insert element Example:
A Python program to insert a new element into a tuple of elements at a specified position.Copied
#inserting a new element into a tuple
names = ('Vishnu', 'Anupama', 'Lakshmi', 'Bheeshma')
print(names)
lst= [input('Enter a new name:')]
new = tuple(lst)
pos = int(input('Enter position no:'))
names1 = names[0:pos-1]
names1 = names1+new
names = names1+names[pos-1:]
print(names)