Python slicing multi-dimensional arrays
A slice represents a part or piece of the array. In the previous sections, we already discussed about slicing in case of single dimensional arrays. Now, we will see how to do slicing in case of multi-dimensional arrays, especially in 2D arrays.
slice two-dimensional array
We take a 2D array with 3 rows and 3 columns as:
a = array([[1, 2, 3], [4, 5, 6], [7, 8, 9]] )
The format of slice operator is: arrayname[start:stop:stepsize]
a[:,:] or a[:] or a[::] will display the following
[[1 2 3]
[4 5 6]
[7 8 9]]
Suppose, we want to display the 0th row from the array, we can write:
a[0,:]
Suppose, we want to display 0th column of the array, we can write:
a[:, 0]
If we want to retrieve only a particular element, then we have to provide both the row and column positions. For example, to retrieve 0th row and 0th column element, we can write:
a[0:1, 0:1]
Similarly, to access the 1st row and 2nd column element, we can write:
a[1:2, 1:2]
let's create a 2D array with 5 rows and 5 columns with elements from 11 to 35.
a = reshape(arange(11, 36, 1), (5,5))
print(a)
a = reshape(arange(11, 36, 1), (5,5))
[[11 12 13 14 15]
[16 17 18 19 20]
[21 22 23 24 25]
[26 27 28 29 30]
[31 32 33 34 35]]
Suppose, we want to display the left top 2 rows and 3 columns, we can write as: print(a[0:2, 0:3])
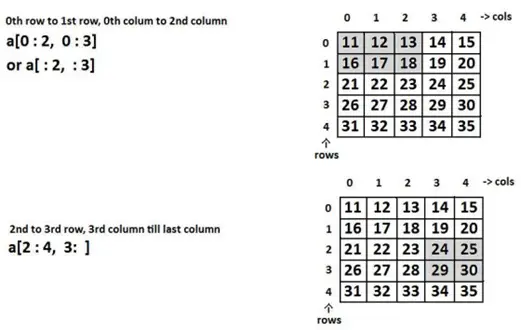
In the same way, we can access the right top 2 rows and 3 columns as: print(a[0:2, 2:])
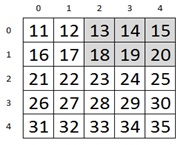
To access the lower right 3 rows and 2 columns, we can write:
print(a[2:, 3:])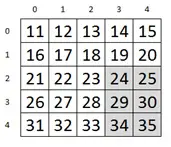