Python Array Copy
In this tutorial, we will learn about how to copy an existing array with the working examples of it.
Array Copy
We can create another array that is same as an existing array. This is done by the view() method. This method creates a copy of an existing array such that the new array will also contain the same elements found in the existing array.
The original array and the newly created arrays will share different memory locations. If the newly created array is modified, the original array will also be modified since the elements in both the arrays will be like mirror images.
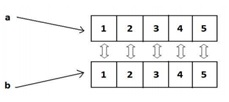
Array view()
from numpy import *
a = arange(1, 6)
b = a.view()
print('Original array:', a)
print('New array:', b)
b[0]=99
print('After modification:')
print('Original array:', a)
print('New array:', b)
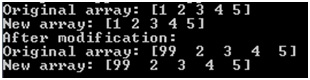
Viewing is nothing but copying only. It is called 'shallow copying ' as the elements in the view when modified will also modify the elements in the original array. So, both the arrays will act as one and the same.
Array deep copy
Suppose we want both the arrays to be independent and modifying one array should not affect another array, we should go for 'deep copying '. This is done with the help of copy() method . This method makes a complete copy of an existing array and its elements. When the newly created array is modified, it will not affect the existing array or vice versa.
Array copy() method
from numpy import *
a = arange(1, 6)
b = a.copy()
print('Original array:', a)
print('New array:', b)
b[0]=99
print('After modification:')
print('Original array:', a)
print('New array:', b)