Python Operators
What is an Operator ?An operator is a symbol that performs an operation. An operator acts on some variables, called operands to get the desired result.
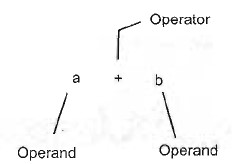
If an operator acts on a single variable, it is called unary operator; if it acts on two variables, it is called binary operator; and if it acts on three variables, then it is called ternary operator.
Operators in Python
- Arithmetic operators
- Assignment operators
- Unary minus operator
- Relational operators
- Logical operators
- Boolean operators
- Bitwise operators
- Membership operators
- Identity operators
Arithmetic Operators
These operators are used to perform basic arithmetic operations like addition, subtraction, division, etc. There are seven arithmetic operators available in Python. Since these operators act on two operands, they are called 'binary operators' also. Let's assume a = 7 and b = 3 and see the effect of various arithmetic operators in the Table
Arithmetic Operators
These operators are used to perform basic arithmetic operations like addition, subtraction, division, etc. There are seven arithmetic operators available in Python. Since these operators act on two operands, they are called 'binary operators' also. Let's assume a = 7 and b = 3 and see the effect of various arithmetic operators in the Table
Operator | Meaning | Example | Result |
---|---|---|---|
+ | Addition operator. Adds two values. | a+b | 10 |
- | Subtraction operator. Subtracts one value from another. | a -b | 4 |
* | Multiplication Operator. Multiplies values on either side of the operator. | a*b | 21 |
/ | Division Operator. Divides left operand by the right operand. | a /b | 2.3333333333333335 |
% | Modulus Operator. Gives remainder of division. | a%b | 1 |
** | Exponent Operator. Calculates exponential power value. a**b gives the value of a to the power of b. | a**b | 343 |
// | Integer Division. This is also called as Floor Division and gives only integer quotient. | a //b | 2 |
When there is an expression that contains several arithmetic operators, we should know which operation is done first and which operation is done next. In such cases, the following order of evaluation is used:
- First parentheses are evaluated.
- Exponentiation is done next.
- Multiplication, division, modulus and floor divisions are at equal priority.
- Addition and subtraction are done afterwards.
- Finally, assignment operation is performed.
Let's take a sample expression: d = (x+y)*z**a//b+c.
Assume the values of variables as: x=1; y=2; z=3; a=2; b=2; c=3.
Then, the given expression d = (1+2)*3**2//2+3 will evaluate as:
- First parentheses are evaluated. d = 3*3**2//2+3.
- Exponentiation is done next. d = 3*9//2+3.
- Multiplication, division, modulus and floor divisions are at equal priority. d = 27//2+3 and then d = 13+3.
- Addition and subtraction are done afterwards. d = 16.
- Finally, assignment is performed. The value 16 is now stored into 'd'. Hence, the total value of the expression becomes 16 which is stored in the variable 'd'.
For order of precedence, just remember this PEMDAS (similar to BODMAS)
Assignment Operators
These operators are useful to store the right side value into a left side variable. These operators are shown in Table. In Table, let's assume the values x = 20, y = 10 and z = 5:
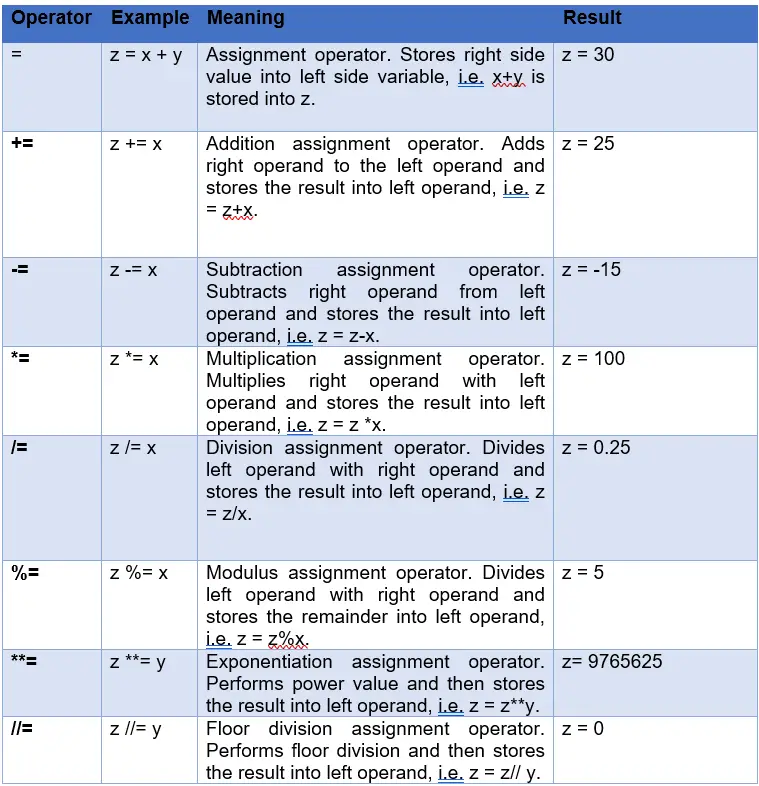
It is possible to assign the same value to two variables in the same statement as:
a=b=1
print(a, b) #will display 1 1
Another example is where we can assign different values to two variables as:
a=1; b=2
print(a, b) #will display 1 2
The same can be done using the following statement:
a, b = 1, 2
print(a, b) #will display 1 2
A word of caution: Python does not have increment operator (++) and decrement operator (--) that are available in C and Java.
Unary Minus Operator
The unary minus operator is denoted by the symbol minus (-). When this operator is used before a variable, its value is negated. That means if the variable value is positive, it will be converted into negative and vice versa.
For example, consider the following statements:
n = 10
print(-n) #displays -10
num = -10
num = - num
print(num) #displays 10
Relational Operators
Relational operators are used to compare two quantities. We can understand whether two values are same or which one is bigger or which one is lesser, etc. using these operators. These operators will result in True or False depending on the values compared, as shown in Table. In this table, we are assuming a =1 and b = 2.
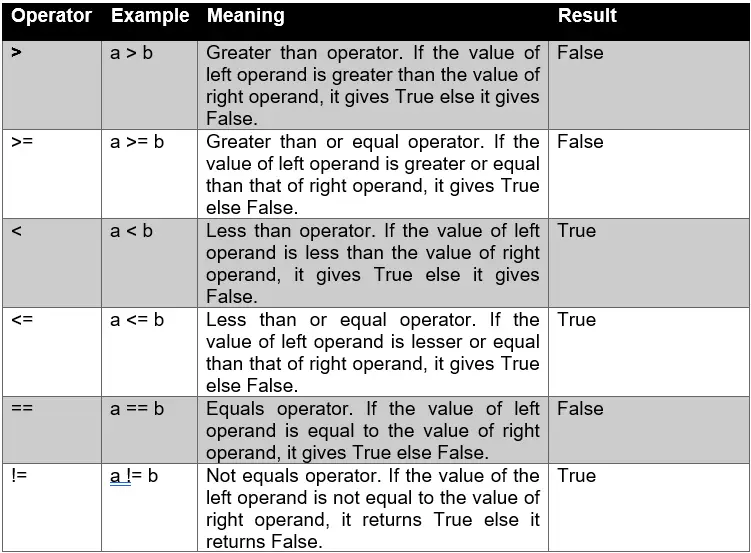
Relational operators are generally used to construct conditions in if statements. For example,
a=1; b=2
if (a>b):
print("Yes")
else:
print("No")
will display 'No'. Observe the expression (a>b) written after if. This is called a 'condition'. When this condition becomes True, if statement will display 'Yes' and if it becomes False, then it will display 'No'. In this case, (1>2) is False and hence 'No' will be displayed.
Relational operators can be chained. It means, a single expression can hold more than one relational operator. For example,
x=15
10<x<20 #displays True
Here, 10 is less than 15 is True, and then 15 is less than 20 is True. Since both the conditions are evaluated to True, the result will be True.
x=15
10>=x<20 #displays False
Here, 10 is greater than or equal to15 is False, and then 15 is less than 20 is True.
10
Solve the following
1<2<3<4 #displays True
1<2>3<4 #displays False
4>2>=2>1 #displays True
Logical Operators
Logical operators are useful to construct compound conditions. A compound condition is a combination of more than one simple condition. Each of the simple condition is evaluated to True or False and then the decision is taken to know whether the total condition is True or False. We should keep in mind that in case of logical operators, False indicates 0 and True indicates any other number. There are 3 logical operators as shown in Table.
Let's take x = 1 and y=2 in this table.
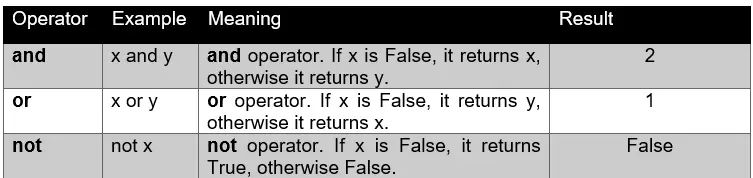
x = 100 y = 200
print(x and y) #will display 200
print(x or y) #will display 100
print (not x) #will display False
Boolean Operators
We know that there are two 'bool' type literals. They are True and False. Boolean operators act upon 'bool' type literals and they provide 'bool' type output. There are three Boolean operators as mentioned in Table.
Let's take x = True and y = False in Table
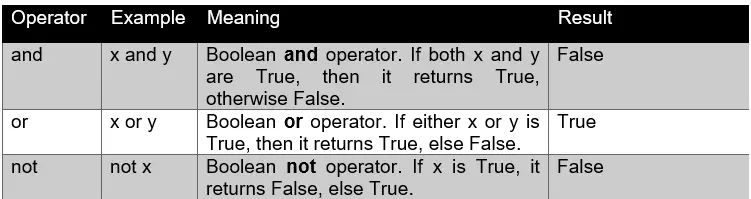