Python slice numpy arrays
Slicing refers to extracting a range of elements from the array. The format of slicing operation is given here:
arrayname[start:stop:stepsize]
The default value for 'start' is 0, for 'stop' is n-1 (n is number of elements) and for 'stepsize' is 1. Counting starts from 0th position. For example, if we write:
a = [10, 11, 12, 13, 14, 15]
a[1:6:2]
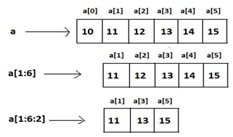

Suppose, we write a[:] or a[::] without specifying anything for start, stop and stepsize, it will extract from 0th element till the end of the array. So, all elements are extracted. Suppose, we write a[2:] , it starts at 2nd element and ends at last element.
slice numpy arrays examples:
What will be the output of the following programCopied
from numpy import *
a = [10, 11, 12, 13, 14, 15]
print(a[-1:4:-1])
Python program to understand slicing operations on arrays.
Copied
from numpy import *
a = arange(10, 16)
print(a)
b = a[1:6:2]
print(b)
b = a[::]
print(b)
b = a[-2:2:-1]
print(b)
b = a[:-2:]
print(b)
Python program to retrieve and display elements of a numpy array using indexing.
Copied
from numpy import *
a = arange(10, 16)
print(a)
a = a[1:6:2]
print(a)
i=0
while(i<len(a)):
print(a[i])
i+=1