Python single Inheritance
Deriving one or more sub classes from a single base class is called 'single inheritance'.
In single inheritance, we always have only one base class, but there can be 'n' number of sub classes derived from it.
Python supports both the inheritances types (single and multiple)
Single Inheritance
Consider the below real time example of python single inheritance
We have a Bank class, which contains a variable and method and this is a single class.
From 'Bank' class we derive 'AndhraBank' and 'StateBank' as sub classes, this concept called as single inheritance.
Consider below image, It is convention that we should use the arrow head towards the base class (i.e. super class) in the inheritance diagrams.
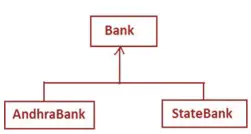
we are deriving two sub classes, named as AndhraBank and StateBank from the single base class, i.e. Bank.
class Bank(object):
cash = 100000000
@classmethod
def available_cash(cls):
print(cls.cash)
In the above Bank class, we have 'cash' variable and a method to display cash variable and will be available to all of it's sub classes.
sub-classes of a Bank Class will be like
class AndhraBank(Bank):
pass
class StateBank(Bank):
cash = 20000000
@classmethod
def available_cash(cls):
print(cls.cash + Bank.cash)
Single Inheritance Example:
Below example contains, Base class and its sub classes, which gives an idea about how to implement or adopt single inheritance in Python.
class Bank(object):
cash = 100000000
@classmethod
def available_cash(cls):
print(cls.cash)
class AndhraBank(Bank):
pass
class StateBank(Bank):
cash = 20000000
@classmethod
def available_cash(cls):
print(cls.cash + Bank.cash)
a = AndhraBank()
a.available_cash()
s = StateBank()
s.available_cash()