Python Multiple Inheritance
Deriving sub classes from multiple (or more than one) base classes is called 'multiple inheritance'. In this type of inheritance, there will be more than one super or base class and there can be one or more sub classes.
All the members of the super classes are by default available to sub classes and the sub classes in turn can have their own members.
The syntax for multiple inheritance is shown in the following statement:
class Subclass(Baseclass1, Baseclass2,...):
Multiple Inheritance example
The best example for multiple inheritance is that parents producing the children and the children inheriting the qualities of the parents.
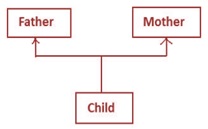
class Father:
def height(self):
print('Height is 6.0 foot')
class Mother:
def color(self):
print('Color is brown')
class Child(Father, Mother):
pass
c = Child()
print('Child\'s inherited qualities:')
c.height()
c.color()
Example:
class A(object):
def __init__(self):
self.a = 'a'
print(self.a)
super().__init__()
class B(object):
def __init__(self):
self.b = 'b'
print(self.b)
class C(A, B):
def __init__(self):
self.c = 'c'
print(self.c)
super().__init__()
o = C()
#Completed
Multiple Inheritance Problems
If the sub class has a constructor, it overrides the super class constructor and hence the super class constructor is not available to the sub class. But writing constructor is very common to initialize the instance variables.
In multiple inheritance, let's assume that a sub class 'C' is derived from two super classes 'A' and 'B' having their own constructors. Even the sub class 'C' also has its constructor. To derive C from A and B, we write:
class C(A, B):
Also, in class C's constructor, we call the super class super class constructor using super().__init__(). Now, if we create an object of class C, first the class C constructor is called. Then super().__init__() will call the class A's constructor.
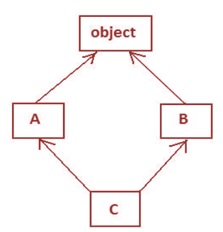
class A(object):
def __init__(self):
self.a = 'a'
print(self.a)
class B(object):
def __init__(self):
self.b = 'b'
print(self.b)
class C(A, B):
def __init__(self):
self.c = 'c'
print(self.c)
super().__init__()
o = C()