Python Exception Handling
An exception is a runtime error which can be handled by the programmer. That means if the programmer can guess an error in the program and he can do something to eliminate the harm caused by that error, then it is called an 'exception'. If the programmer cannot do anything in case of an error, then it is called an 'error' and not an exception.
All exceptions are represented as classes in Python. The exceptions which are already available in Python are called 'built-in' exceptions. The base class for all built-in exceptions is 'BaseException ' class. From BaseException class, the sub class 'Exception ' is derived. From Exception class, the sub classes 'StandardError ' and 'Warning ' are derived.
All errors (or exceptions) are defined as sub classes of StandardError . An error should be compulsorily handled otherwise the program will not execute. Similarly, all warnings are derived as sub classes from 'Warning ' class. A warning represents a caution and even though it is not handled, the program will execute. So, warnings can be neglected but errors cannot be neglected.
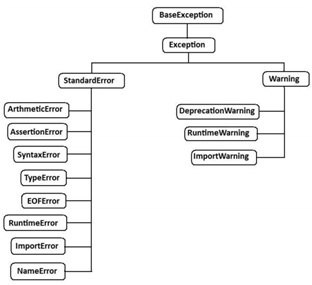
Exception Handling
The purpose of handling errors is to make the program robust. The word 'robust' means 'strong'. A robust program does not terminate in the middle. Also, when there is an error in the program, it will display an appropriate message to the user and continue execution. Designing such programs is needed in any software development. For this purpose, the programmer should handle the errors. When the errors can be handled, they are called exceptions. To handle exceptions, the programmer should perform the following three steps:
Step 1: The programmer should observe the statements in his program where there may be a possibility of exceptions. Such statements should be written inside a 'try' block. A try block looks like as follows:
try:
statements
The greatness of try block is that even if some exception arises inside it, the program will not be terminated. When PVM understands that there is an exception, it jumps into an 'except' block.
Step 2: The programmer should write the 'except' block where he should display the exception details to the user. This helps the user to understand that there is some error in the program. The programmer should also display a message regarding what can be done to avoid this error. Except block looks like as follows:
except exceptionname:
statements #these statements form handler
The statements written inside an except block are called 'handlers' since they handle the situation when the exception occurs.
Step 3: Lastly, the programmer should perform clean up actions like closing the files and terminating any other processes which are running. The programmer should write this code in the finally block. Finally block looks like as follows:
finally:
statements
The specialty of finally block is that the statements inside the finally block are executed irrespective of whether there is an exception or not. This ensures that all the opened files are properly closed and all the running processes are properly terminated. So, the data in the files will not be corrupted and the user is at the safe-side.
Performing the above 3 tasks is called 'exception handling'.
Exception Handling Example
#an exception handling example
try:
f = open("myfile", "w")
a, b = [int(x) for x in input("Enter two numbers: ").split()]
c = a/b
f.write("writing --.2f into myfile" --c)
except ZeroDivisionError:
print('Division by zero happened')
print('Please do not enter 0 in denominator')
finally:
f.close()
print('File closed')
From the preceding output, we can understand that the 'finally' block is executed and the file is closed in both the cases, i.e. when there is no exception and when the exception occurred. In the previous discussion, we used try-catch-finally to handle the exception. However, the complete exception handling syntax will be in the following format:
try:
statements
except Exception1:
handler1
except Exception2:
handler2
else:
statements
finally:
statements
The following points are noteworthy:
- A single try block can be followed by several except blocks.
- Multiple except blocks can be used to handle multiple exceptions.
- We cannot write except blocks without a try block.
- We can write a try block without any except blocks.
- Else block and finally blocks are not compulsory.
- When there is no exception, else block is executed after try block.
- Finally block is always executed.