python class creation
A python class is created with the keyword class and then writing the Classname. After the Classname, 'object' is written inside the Classname. This 'object' represents the base python class name from where all classes in Python are derived. Even our own python classes are also derived from 'object' class. Hence, we should mention 'object' in the parentheses. Please note that writing 'object' is not compulsory since it is implied.
python class syntax
class Student:
def __init__(self):
self.name = 'Ram'
self.age = 20
self.marks = 900
def talk(self):
print('Hi, I am ', self.name)
print('My age is', self.age)
print('My marks are', self.marks)
In python, we cannot declare variables, we have written the variables inside a special method, i.e.__init__()
This method is useful to initialize the variables. Hence, the name 'init'. The method name has two underscores before and after. This indicates that this method is internally defined and we cannot call this method explicitly.
Observe the parameter 'self' written after the method name in the parentheses. 'self' is a variable that refers to current class instance .
When we create an instance for the Student class, a separate memory block is allocated on the heap and that memory location is by default stored in 'self'.
The instance contains the variables 'name', 'age', 'marks' which are called instance variables. To refer to instance variables, we can use the dot operator notation along with self as: 'self.name ', 'self.age ' and 'self.marks '.
The methods that act on instances (or objects) of a class are called instance methods . Instance methods use 'self' as the first parameter that refers to the location of the instance in the memory. Since instance methods know the location of instance, they can act on the instance variables.
To create an instance, the following syntax is used:
instancename = Classname()
So, to create an instance (or object) to the Student class, we can write as:
s1 = Student()
Here, 's1' is nothing but the instance name. When we create an instance like this, the following steps will take place internally:
- First of all, a block of memory is allocated on heap. How much memory is to be allocated is decided from the attributes and methods available in the Student class.
- After allocating the memory block, the special method by the name '__init__(self)' is called internally. This method stores the initial data into the variables. Since this method is useful to construct the instance, it is called 'constructor'.
- Finally, the allocated memory location address of the instance is returned into 's1' variable. To see this memory location in decimal number format, we can use id() function as id(s1).
Now, 's1' refers to the instance of the Student class. Hence any variables or methods in the instance can be referenced by 's1' using dot operator
s1.name #this refers to data in name variable, i.e. Vishnu
s1.age #this refers to data in age variable, i.e. 20
s1.marks #this refers to data in marks variable, i.e. 900
s1.talk() #this calls the talk() method
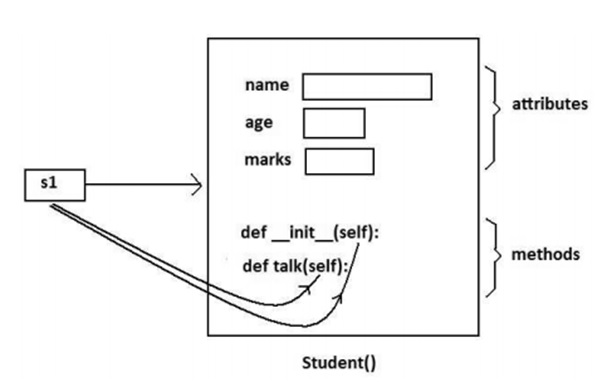
python class example
A Python program to define Student python class and create an object to it. Also, we will call the method and display the student's details.
#instance variables and instance method
class Student:
#this is a special method called constructor.
def __init__(self):
self.name = 'Vishnu'
self.age = 20
self.marks = 900
#this is an instance method.
def talk(self):
print('Hi, I am', self.name)
print('My age is', self.age)
print('My marks are', self.marks)
#create an instance to Student class.
s1 = Student()
#call the method using the instance.
s1.talk()
Here, we used the 'self ' variable to refer to the instance of the same class. Also, we used a special method '__init__(self) ' that initializes the variables of the instance. Let's have more clarity on these two concepts.