Python namespaces
In this tutorial, we will learn about what is namespace, types of namespaces and its modification along with the example.
Namespace
A namespace represents a memory block where names are mapped (or linked) to objects. Suppose we write:
n = 10
Here, �n� is the name given to the integer object 10. Please recollect that numbers, strings, and lists� are all considered as objects in Python. In the above name �n� is linked to 10 in the namespace.
Class and instance namespaces
A class maintains its own namespace, called �class namespace�. In the class namespace, the names are mapped to class variables. Similarly, every instance will have its own name space, called �instance namespace� In the instance namespace, the names are mapped to instance variables.
class namespace:
Modifying class namespace can be done in the following way.
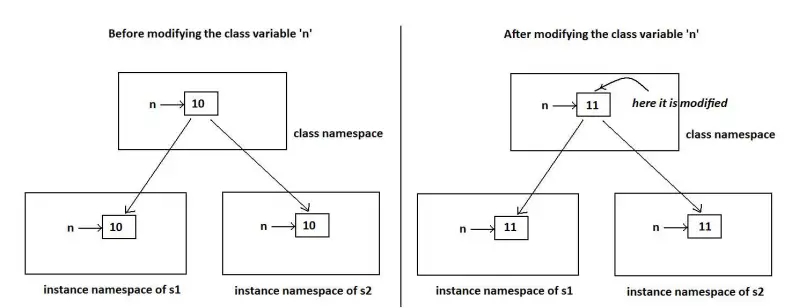
class Student:
n=10
print(Student.n)
Student.n+=1
print(Student.n)
instance namespace:
Modifying class variable in the instance namespace can be done in the following way.
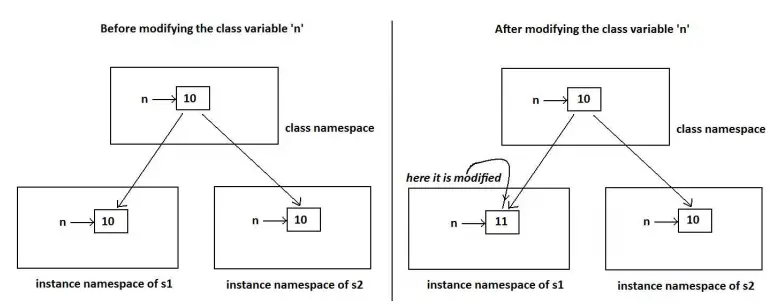
class Student:
n=10
s1 = Student()
s2 = Student()
print(s1.n) #displays 10
s1.n+=1 #modify it in s1 instance namespace
print(s1.n) #displays 11
print(s2.n)
Conclusion:
In this tutorial, we have learned about what is namespace in python and types of namespaces and modifying those.