Python tkinter Menu
A menu represents a group of items or options for the user to select from. For example, when we click on 'File' menu, it may display options like 'New', 'Open', 'Save', etc.We can select any option depending on our requirements.'New', 'Open', 'Save' - these options are called menu items.Thus, a menu is composed of several menu items. Similarly, 'Edit' is a menu with menu items like 'Cut', 'Copy', 'Paste', etc. Generally, we see menus displayed in a bar, called menu bar.
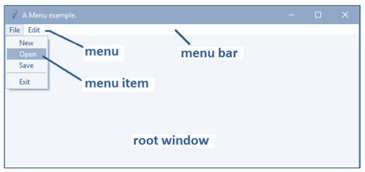
tkinter Menu
To create a menu, we should use the following steps:
- First of all, we should create a menu bar as a child to root window. This is done using Menu class as: menubar = Menu(root)
- This menu bar should be attached to the root window using config() method as: root.config(menu=menubar)
- The next step is to create a menu with a group of menu items. For this purpose, first of all we should create Menu class object as:
filemenu = Menu(root, tearoff=0)
Here, 'filemenu' is the Menu class object. The option 'tearoff' can be 0 or 1.When this option is 1, the menu can be torn off.In this case, the first position (position 0) in the menu items is occupied by the tear-off element which is a dashed line. If this option value is 0, then this dashed line will not appear and the menu items are displayed starting from 0th position onwards.The next step is to add menu items to the filemenu object using the add_command() method as:
filemenu.add_command(label="New", command=donothing)
When we want to display a horizontal line that separates a group of menu items from another group of menu items, we can use the add_separator() method, as given in the following statement: filemenu.add_separator() After adding all menu items to filemenu object, we should give it a name and add it to menu bar using add_cascade() method as: menubar.add_cascade(label="File", menu=filemenu) The 'menu' option tells that the 'File' menu is composed of all menu items that are added already to filemenu object.
tkinter Menu example
A Python program to create a menu bar and adding File and Edit menus with some menu items
#to display a menubar with File and Edit menus
from tkinter import *
class MyMenuDemo:
def __init__(self, root): #create a menubar self.menubar = Menu(root) #attach the menubar to the root window root.config(menu=self.menubar) #create file menu self.filemenu = Menu(root, tearoff=0) #create menu items in file menu self.filemenu.add_command(label="New", command=self.donothing) self.filemenu.add_command(label="Open", command=self.donothing) self.filemenu.add_command(label="Save", command=self.donothing) #add a horizontal line as separator self.filemenu.add_separator() #create another menu item below the separator self.filemenu.add_command(label="Exit", command=root.destroy) #add the file menu with a name 'File' to the menubar self.menubar.add_cascade(label="File", menu=self.filemenu) #create edit menu self.editmenu = Menu(root, tearoff=0)
#create menu items in edit menu self.editmenu.add_command(label="Cut", command=self.donothing) self.editmenu.add_command(label="Copy", command=self.donothing) self.editmenu.add_command(label="Paste",command=self.donothing) #add the edit menu with a name 'Edit' to the menubar self.menubar.add_cascade(label="Edit", menu=self.editmenu)
def donothing(self):
pass #create root window
root = Tk() #title for the root window
root.title("A Menu example.") #create object to our class
obj = MyMenuDemo(root) #define the size of the root window
root.geometry('600x350') #handle any events
root.mainloop()