Python OOPS Advantages
Software development is a team effort. Several programmers will work as a team to develop software. When a programmer develops a class, he will use its features by creating an instance to it. When another programmer wants to create another class which is similar to the class already created, then he need not create the class from the scratch. He can simply use the features of the existing class in creating his own class.
OOPS Advantages with examples:
Lets consider the below real time advantage of the python oops as compare to the normal flow.
A programmer in the software development is creating Teacher class with setter() and getter() methods. Then he saved this code in a file 'teacher.py'.
class Teacher:
def setid(self, id):
self.id = id
def getid(self):
return self.id
def setname(self, name):
self.name = name
def getname(self):
return self.name
def setaddress(self, address):
self.address = address
def getaddress(self):
return self.address
def setsalary(self, salary):
self.salary = salary
def getsalary(self):
return self.salary
When the programmer wants to use this Teacher class that is available in teacher.py file, he can simply import this class into his program and use it as shown below:
from teacher import Teacher
#create instance
t = Teacher()
#store data into the instance
t.setid(10)
t.setname('Prakash')
t.setaddress('H.No.-29, Neeruganti Street, Anantapur')
t.setsalary(25000.50)
#retrieve data from instance and display
print('id=', t.getid())
print('name=', t.getname())
print('address=', t.getaddress())
print('salary=', t.getsalary())
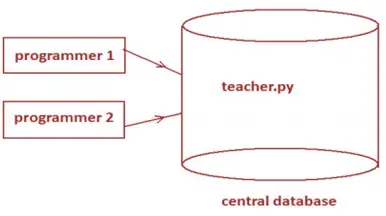
Now, another programmer in the same team wants to create a Student class. He is planning the Student class without considering the Teacher class as shown below
A Python program to create Student class and store it into student.py module.
#this is Student class, save it as stu.py
class Student:
def setid(self, id):
self.id = id
def getid(self):
return self.id
def setname(self, name):
self.name = name
def getname(self):
return self.name
def setaddress(self, address):
self.address = address
def getaddress(self):
return self.address
def setmarks(self, marks):
self.marks = marks
def getmarks(self):
return self.marks
Now, the second programmer who created this Student class and saved it as student.py can use it whenever he needs.
A Python program to use the Student class which is already available in stu.py
#save this code as inh.py
from stu import Student
s = Student()
s.setid(100)
s.setname('Rakesh')
s.setaddress('H.No.-231, Old Town, Anantapur')
s.setmarks(970)
print('id=', s.getid())
print('name=', s.getname())
print('address=', s.getaddress())
print('marks=', s.getmarks())
If we compare the Teacher class and the Student classes, we can understand that 75-- of the code is same in both the classes. That means most of the code being planned by the second programmer in his Student class is already available in the Teacher class. Then why doesn't he use it for his advantage? Our idea is this: instead of creating a new class altogether, he can reuse the code which is already available.
A Python program to create Student class by deriving it from the Teacher class.
#save it as student.py
from teacher import Teacher
class Student(Teacher):
def setmarks(self, marks):
self.marks = marks
def getmarks(self):
return self.marks
class Student(Teacher):
This means the Student class is derived from Teacher class. Once we write like this, all the members of Teacher class are available to the Student class. Hence we can use them without rewriting them in the Student class.
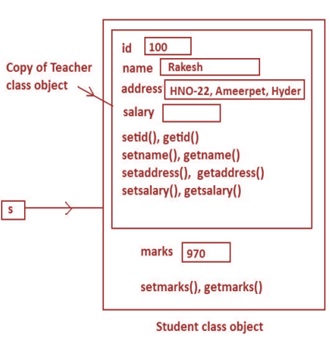