Java OOPs Concepts
In this tutorial, we will learn about OOPs, its core concepts and explanation of each along with real time scenarios where OOPs and its concepts can be used.
OOPs Concepts
OOPs stands for Object Oriented Programming and its fundamental concept is to deal with objects and classes, objects are considered as real entities. Assume there is a batch of students which are studying 10th standard, 10th standard is not a real entity but students are real entities who belongs to 10th standard.
In the consideration of students and 10th standard, students are real entities considered as objects in the OOPs and 10th standard is considered as class. So, object can anything that really exists in the world and will be distinguished with others.
Class is a user defined blue print or prototype from which objects are created, in students and 10th standard scenario standard is a class. Class will be only one but objects can be many, each object will have its own features. In student's case their name, address and marks will be different but all belongs to 10th standard.
The following are the major concepts of the OOPs.
- Encapsulation
- Abstraction
- Inheritance
- Polymorphism
Encapsulation:
Encapsulation is a concept, which refers to binding of the data members and methods which operate on data members in a single class. The data and methods will not be exposed to the outside of the class this in turns provides security to the data and helps to avoid manipulating the data.
One such real example can be an online banking transaction, data of the bank can be accessed or modified inside of the bank website not outside of it.
Encapsulation can be achieved in the Java with two steps.
- Declaring all the data operating methods inside a single class
- Restricting the access to the operating methods by limiting its access.
Abstraction:
Meaning of abstract is an idea of something which is not physically exists, similarly abstraction in the oops is declaring something expected a desired outcome but steps to achieve desired results will not be mentioned or leaving it to the developer to achieve desired outcome.
Abstraction concept of oops helps to achieve the following.
- Reusability
- Customization
Reusability:
Main goal of abstraction is DRY and DRY stands for "Do Not Repeat Yourself" having a block of code does which performs the same operations from different places will make no sense.
Abstraction suggests to merge all those blocks in one place, which in turns to avoid the duplication of the code. By this way reusability can be achieved and advantages of do it as follows.
- Clean code and easier to read and change.
- Makes life simple for testers in terms of reduction in test cases.
- Efficiency of developers will be improved.
Customization:
Customization can be done based on necessity of the business requirements. Assume, there is banking application in which a module of deductions from the balance given to you to write.
Generally, in banks customer can have multiple type of accounts, for simplicity consider only two accounts.
- Savings Account
- Credit Account
Considering saving and credit account deductions will not be the same, saving account amount can be deducted if there is a sufficient balance unlike the credit account.
If we do not follow abstraction will end up writing multiple blocks of code not just for deductions and it increases the complexity.
To handle this kind of scenarios, first need to know what data will not be changed and what gets changed in this case customer details will not be changed like name, address, email, and phone number but way balance gets deducted will be changed based on account type.
By implementing all the unchanged data in one class and logic of deduction in other class, implement a master class with the implementations of unchanged data and leave deduction method as abstraction.
Later savings and credit account classes will implement the deduction method based on account type.
In java abstraction will be achieved by using the keyword abstract at class level and at method level and sub class or classes will implement abstract methods basis on their requirements.
Inheritance:
Meaning of inheritance is inheriting either property or money as heir, in the oops also inheritance defines the same way to follow while building an application.
Parent will be a super class and child classes will be heir of super class, while child class will have an access to the super class methods and data members.
In java, inheritance can be achieved by extending its parent or super class using keyword extends, after extending by default all the variables and methods will be accessible to the child class.
Inheritance have one famous well known problem is "diamond problem", diamond problem is the ambiguity of deciding which method to execute when child class extends two classes but both the classes have same method with same signature.
Consider an example of a child class which extends both Mother and Father.
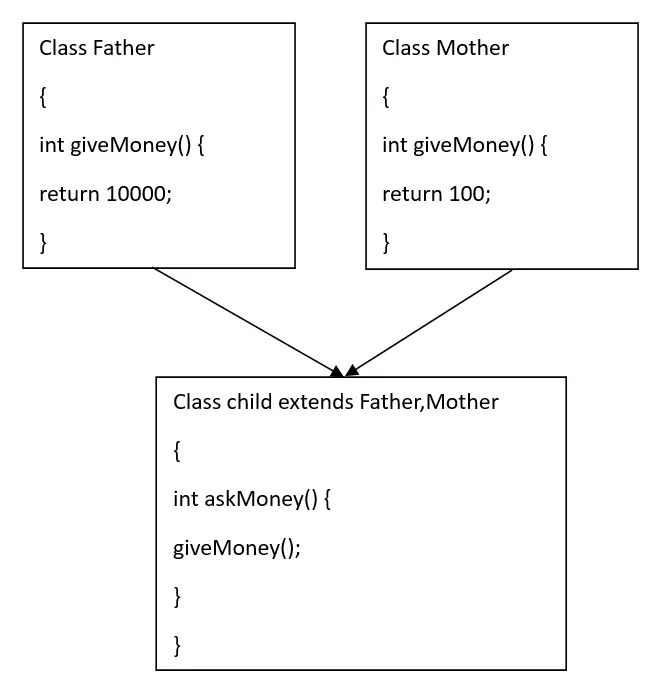
Mother and Father will become super class for the child class, now the both parents have method named as giveMoney() with the same signature. In these conditions compiler will not know for which super class method to be executed and this ambiguity known as "Diamond Problem".
Until Java 8, java never exposed to the diamond problem because it was not supporting multiple inheritance. From Java 8 onwards interface can have default methods, if a child interface extends multiple interfaces of having the same signature one or more default methods, then child should contain default method implementation in it otherwise compilation for that child interface will lead to an error or fails.
Polymorphism:
Polymorphism is a concept of having same name but exists in different forms, consider an example of numbers and numbers can be a decimal or integer at the end all are numbers only, in the similar way polymorphism in OOPS will have the same name but will be accepting different forms( can be integer or decimal).
consider we are performing an addition of two numbers in calculator, if we open any calculator will have an option to give input as an integer or decimal will display the results.
But in computer programming language( at least in Java) integers will be a different type and decimal will be different types but all are numbers only, the underlying operation needs to perform addition only.
Will declare addition of two numbers methods with different combinations like following.
- Both are integers
- Both are decimals.
- First number is a decimal and second number is an integer.
- First number is an integer and second number is a decimal.
Java allows to have the same method name with different types of parameters as arguments.
Importance of OOPs
- Improves the reusability and reduces redundancy of the code.
- Flexible to handle customization in different layer without breaking of DRY principle.
- Through inheritance will have direct access or control of super class members or methods.
- Objects will have different properties, like real world entities.
Conclusion :
In this tutorial, we have learned about OOPs concepts and its principles, Diamond problem in inheritance and importance of oops.