Java Data Types
In this tutorial, we will learn about what data types are present in java, declaration of data type and an example of one customized a user defined data type.
What is a data type in Java
Before declaring a variable, it requires to declare its type. Type specifies which kind of data it can be holding and type of a data can be number or character or complex type. Based on the declaration of the type subsequent memory will be allocated for that specific variable.
Types of data types:
In Java, data types are classified broadly two types. One is being the primitive type and other being the user defined type.
- Primitive types
- Complex or user-defined types
Primitive types
There are 8 primitives are available, each primitive type specifies what type of data it can hold or store in the memory in other words primitives are classified into 8 types based on the type of data holding by those with range.
Primitive will have the size limit and it can hold the data up to a certain range only. Before declaring as primitive one should have an idea about size of the data before declaring.
Byte Type:
The byte type is an 8-bit signed two's complement integer, this type can hold min value of -128 and max value of 127. While writing an application where memory is bigger constraint can help to reduce the memory usage, if the value falls under its limit.
Declaration of byte type:
Byte data type variable value can be declared in numeric or binary format but the value should be within range of byte.
byte b = 36;
byte bits = 0b00100100;
In the above, we declared byte type one with numeric format other with binary format. While specifying binary format it should be prefix with '0b'
short type :
The short data type is a 16-bit signed two's complement integer, this type can hold min value of -32,768 and max value of 32,767. It follows the same principle as byte and can be used where memory is constraint.
Declaration of short type:
short data type variable value can be declared in numeric or binary format but the value should be within its range.
short shrt = 36;
short shInbinary = 0b00100100;
int Type:
The int type is a 32-bit signed two's complement integer, this type can hold min value of -2,147,483,648 and max value of 2,147,483,647.
Declaration of int type:
int data type variable value can be declared in numeric or binary format but the value should be within its range.
int intNumeric = 36;
int intbinary = 0b00100100;
long Type:
The long type is a 64-bit signed two's complement integer, this type can hold min value of -9,223,372,036,854,775,808 and max value of 9,223,372,036,854,775,807.
Declaration of long type:
long data type variable value should be declared with the postfix L or l otherwise will be considered as int type while value with in the range of int type outside of int range will cause a compile time error.
long longVariable = 9223372036854775807L;
It is recommended to use L instead of l otherwise it is hard to distinguish it.
float type :
The float type is a single-precision 32-bit floating point follows IEEE-754 standard, IEEE-754 describes floating point formats. This type can hold up to 7 decimal digits of precision.
Declaration of float type:
float data type variable value should be declared with the postfix F or f otherwise will be considered as double type.
float floatVariable = 922.33721F;
double type :
The double type is a double-precision 64-bit floating point follows IEEE-754 standard, IEEE-754 describes floating point formats. This type can hold up to 15 decimal digits of precision.
Declaration of double type:
double data type variable value declaring with the postfix D or d is optional.
double doubleVariable = 922.33721345123;
Size, range, and default values of primitives shown in the following table.
S.No | Data Type | Size | Range | Default Value |
---|---|---|---|---|
1 | byte | 1 byte | store numbers from -128 to 127 | 0 |
2 | short | 2 bytes | stores numbers from -32,768 to 32,767 | 0 |
3 | int | 4 bytes | stores numbers from -2,147,483,648 to 2,147,483,647 | 0 |
4 | long | 8 bytes | stores numbers from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 | 0L |
5 | float | 4 bytes | stores decimal numbers, stores up to 7 decimal digits of precision. | 0.0f |
6 | double | 8 bytes | stores decimal numbers, stores up to 15 decimal digits of precision. | 0.0d |
7 | char | 2 bytes | Stores single character | '\u0000' |
8 | boolean | 1 bit | stores either true or false | false |
Complex or user-defined data types:
As we learned, datatype can be declared as user defined type and will consider one example as such and write an example of custom datatype.
While writing an application, it requires to have user-defined type. Suppose if we are writing a program to display student details. Student will be having name, studying class, group , address, and school name. To handle this requirement custom data type is required.
Student will be considered as one example, in which student will have name, class, group, address and institute name.
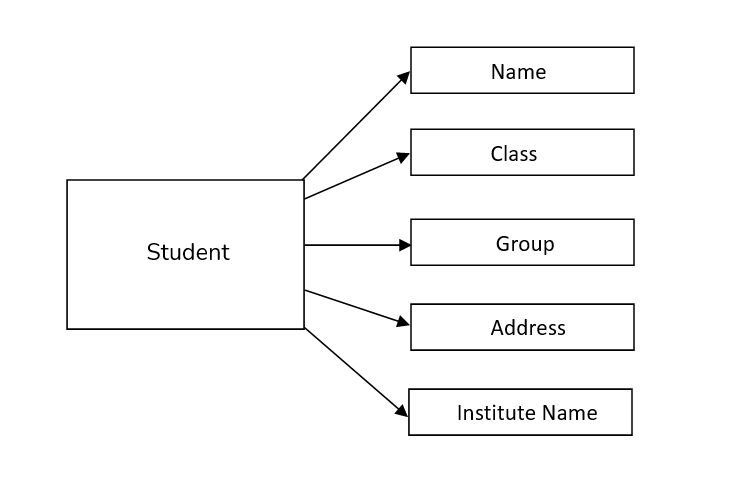
We do not have inbuilt type for Student, it requires to customize this type.
Following example will show how to customize the student datatype.
Complex or user-defined type example:
The following is one of the example of custom (or) user-defined type.
class StudentDataType {
public String studentName;
public String studentClass;
public String studentGroup;
public String studentAddress;
public String studentInstituteName;
public StudentDataType(String studentName, String studentClass,
String studentGroup, String studentAddress, String studentInstituteName) {
this.studentName = studentName;
this.studentClass = studentClass;
this.studentGroup = studentGroup;
this.studentAddress = studentAddress;
this.studentInstituteName = studentInstituteName;
}
public String getStudentName() {
return this.studentName;
}
public String getStudentClass() {
return this.studentClass;
}
public String getStudentGroup() {
return this.studentGroup;
}
public String getStudentAddress() {
return this.studentAddress;
}
public String getStudentInstituteName() {
return this.studentInstituteName;
}
}
public class Student {
public static void main(String[] args) {
StudentDataType student = new StudentDataType("Varun", "8th Class",
"GROUP-A", "Delhi,India", "Higher School of Delhi");
System.out.println("Student Name :"+student.getStudentName());
System.out.println("Student Class :"+student.getStudentClass());
System.out.println("Student Group :"+student.getStudentGroup());
System.out.println("Student Address :"+student.getStudentAddress());
System.out.println("Student Institute name :"
+student.getStudentInstituteName());
}
}
Output
Student Name :Varun
Student Class :8th Class
Student Group :GROUP-A
Student Address :Delhi,India
Student Institute name :Higher School of Delhi
Conclusion
In this tutorial, we have learned about the java data types of both primitive and custom with a working example of it.