Java for loop
In this tutorial, we will learn about for loop of java, syntax, and a working example along with new enhanced for loop including an example of nested for loop.
What is a for loop
Looping is a concept in the computer programming, which allows programmers or developers to execute a block of statements repeatedly until the looping condition not satisfied. Looping concept helps to perform iterate a group objects or elements and perform operations individually on them, for loop is one of such loops and can be used to iterate objects and perform operations among those objects individually.
For loop:
In Java, for loop is used to execute a block of statements in a loop manner based on the condition of the loop. Consider an example of cooking vegetables on a stove, we will be checking few times whether its properly boiled or need to cook some more and will be checking until its boiled nothing loop of testing until its cooked. To handle this kind of requirement, looping is the best suit to fulfil it.
From Java 5 onwards a new syntax of for loop introduced to iterate arrays and lists, before this new enhanced for loop iterating of list usually done by while loop and arrays iteration will be done by incrementing the index of array using both for loop and while loop.
Syntax of for loop:
The following is the syntax of the for loop for standard iterations.
for(stmt1;stmt2;stmt3) {
//statements inside loop
}
Syntax of new for loop:
The following is the syntax of new for loop, which allows to iterate group of elements and arrays also with out being checked its size unlike old for loop. This new for loop introduced on Java 5.
for(Object obj: objectList) {
//statements inside loop
}
Example of old for loop:
public class ForLoopExample {
public static void main(String[] args) {
for(int i=1;i<=5;i++) {
System.out.println(i);
}
}
}
Output:
1
2
3
4
5
After executing the example program, numbers will be printed from 1 to 5 as shown above. Explanation for the example as following,
- In statement1, initialized and declared a variable i with value as 1 type as int.
- In statement2, checks value of i value should be less than or equals to 5.
- Increments i value with post increment using ++.
- i value as 1 which is less than 5, loop gets executed and prints i value.
- In second execution i value increments by 1 and becomes 2 which is less than 5 and loop gets executed and prints i value.
- Loop continues to execute until the i value becomes greater than 5.
For every single execution of loop, i value incremented by 1 and thereby 5 times loop gets executed.
The next example will be done using new syntax of for and will iterate an array of elements type is a int.
Example of new for loop:
public class ForWithNewSyntax {
public static void main(String[] args) {
int[] array = {1,2,3,4,5,6,10,7};
for (int arr : array)
{
System.out.println(arr);
}
}
}
Output:
1
2
3
4
5
6
10
7
After executing the example, the above lines get printed, the following explanation for the output of example.
- Declared an array of int type and assigned random numbers to it.
- Declared for loop to iterate array elements of variable array and assign each element of array to a variable arr.
- For each iteration, element of array assigned to arr variable by incrementing index of array variable.
- Above loop gets executed until the size of the array is less than index of array.
- Total of 8 times loop gets executed and prints array elements.
Following important points to remember about the new for loop syntax.
- New for loop syntax popularly referred as for-each loop.
- New syntax of for loop will be converted into old for loop syntax after compilation of it.
The following is the screen shot of decompiled for each block of the example.
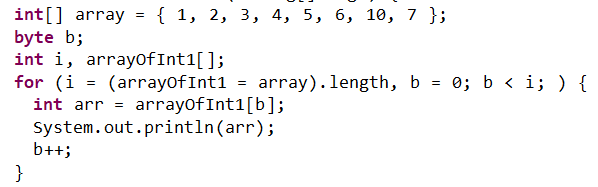
Nested for loop
A for loop embedded into another for loop referred as nested for loop, these nested loops can be used to handle the two or more-dimensional arrays.
In the following example, we will print a * symbol in a right-angle triangle shape.
Nested loop example:
public class NestedForLoopExample {
public static void main(String[] args) {
for (int i=0;i<5;i++)
{
for(int j=0;j<=i;j++)
{
System.out.print("*");
}
System.out.println("\n");
}
}
}
Output:
*
**
***
****
*****
After executing the example of nested for loop will print the above lines of output, explanation for the output and example as follows.
- We have two for loops, one is inside of another loop.
- Top one will execute 5 times.
- Inside loop will execute until the j variable value greater than parent loop variable i value.
- While i value is 1 print one time * symbol.
- When i value is 2 print two times * symbol and goes till i value becomes not less than 5.
- The above example indicates an array of two dimensional. Array[i][j] with the column and row size as i, j respectively.
Conclusion:
In this tutorial, we have learned about the for loop with syntax with working example and step by step explanation of handling two dimensional arrays with nested for loop.